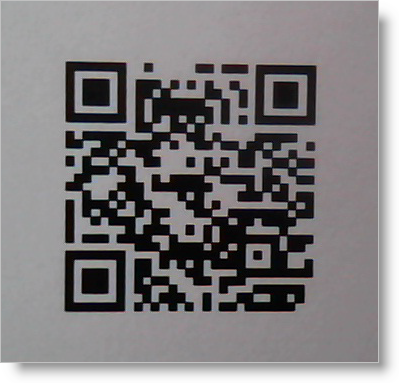
This topic introduces you to the Infragistics Barcode Reader™ library and explains how to work with it in C# and VB. Its properties, classes and methods are explained too, as well as the optional use of the Symbology enumeration. At the end, full code samples are provided in a separate section. The content of the topic is organized in sections as follows:
List of the supported symbologies.
Implementing the Image Decoding Functionality
Step-by-step instructions.
Using the Infragistics Barcode Reader
Properties, classes, methods, enumeration.
Instantiating a new Barcode Reader object and configuring its properties.
Infragistics Barcode Reader is a library for decoding barcodes from an image.
The following procedure implements the image decoding functionality, that is, the capability to scan an image for a barcode(s).
Add the required assemblies.
Add the following NuGet package to your application. For more information on setting up the NuGet feed and adding NuGet packages, you can take a look at the following documentation: NuGet Feeds.
Infragistics.WPF.BarcodeReader
Add the required namespaces.
Place using/Imports in your code-behind:
In Visual Basic:
Imports Infragistics.Controls.Barcodes
In C#:
using Infragistics.Controls.Barcodes;
Create a new Barcode Reader instance, attach an event handler, and start the decoding process.
Add a new instance of the Barcode Reader in your code-behind. When the Barcode Reader decodes a barcode or finishes scanning an image, the DecodeComplete event is raised as many times as barcode symbols are recognized in the image. Attach an event handler for the DecodeComplete event and then start the decoding process using either the Decode or DecodeAsync (thread-safe) method.
In Visual Basic:
Dim barcodeReader As New BarcodeReader()
AddHandler barcodeReader.DecodeComplete, New EventHandler(Of ReaderDecodeArgs)(AddressOf BarcodeReader_DecodeComplete)
barcodeReader.Decode(aBitmapSourceObject)
In C#:
BarcodeReader barcodeReader = new BarcodeReader();
barcodeReader.DecodeComplete += new EventHandler<ReaderDecodeArgs>(BarcodeReader_DecodeComplete);
barcodeReader.Decode(aBitmapSourceObject);
Process the decoded data.
Handle the decoded data in the DecodeComplete event handler. Note that the DecodeComplete event is raised for every barcode symbol found in the specified image after the Barcode Reader finishes scanning the image.
In Visual Basic:
Private Sub BarcodeReader_DecodeComplete(sender As Object, e As ReaderDecodeArgs)
If e.SymbolFound Then
Dim result As String = e.Symbology & " " & e.Value
End If
End Sub
In C#:
private void BarcodeReader_DecodeComplete(object sender, ReaderDecodeArgs e)
{
if (e.SymbolFound)
{
string result = e.Symbology + " " + e.Value;
}
}
The Barcode Reader library has the following properties:
MaxNumberOfSymbolsToRead – the maximum number of barcodes that are expected on the image (-1 for all, default: 1, recommended: 1÷5)
When this threshold is reached, the Barcode Reader stops the decoding. A low number of barcodes for recognition will increase the application’s performance. When -1 is assigned, the Barcode Reader will retrieve symbols until all of them are found.
The picture below demonstrates a scanned image (retrieved by the FilteredImage property) with the MaxNumberOfSymbolsToRead set to 1 – only one symbol is recognized (the one with the green rectangle over it – Code 128):
Figure 2: Effect of the MaxNumberOfSymbolsToRead setting on the barcodes retrieved
MinSymbolSize – minimum recognizable symbol size in pixels (width and height for linear barcodes only) –(default: -1, recommended: a value proportional to the image size, e.g. 50 for an image with 500px height)
The default value of -1 means that the minimum recognizable symbol size is to be calculated internally depending proportionally on the scanned image size. The lower the MinSymbolSize value, the more zones the image will be divided into (this enables recognizing of small symbols but has a negative effect on performance), the higher is just the opposite (fewer zones, symbols should be bigger, better performance). Note that if a symbols’ size in pixels is less than the specified in MinSymbolSize, the image might not be decoded.
BarcodeOrientation – orientation of the scanned barcodes (linear barcodes only)
Horizontal
Vertical
Unspecified (default)
Unspecified orientation means that a barcode is to be decoded regardless of its orientation.
FilteredImage - an image with the recognized barcode symbols. (Figure 3: A filtered barcode image) Used only with the Decode method.
Figure 3: A filtered barcode image
The following code samples demonstrate configuring the Barcode Reader properties as follows:
maximum number of symbols to read: 3
minimum symbol size: 20 pixels
horizontal orientation
In Visual Basic:
Dim barcodeReader As New BarcodeReader()
barcodeReader.MaxNumberOfSymbolsToRead = 3
barcodeReader.MinSymbolSize = 20
barcodeReader.BarcodeOrientation = SymbolOrientation.Horizontal
In C#:
BarcodeReader barcodeReader = new BarcodeReader();
barcodeReader.MaxNumberOfSymbolsToRead = 3;
barcodeReader.MinSymbolSize = 20;
barcodeReader.BarcodeOrientation = SymbolOrientation.Horizontal;
The DecodeAsync method allows an image to be decoded thread-safely – several images can be decoded simultaneously in different threads:
In Visual Basic:
Deployment.Current.Dispatcher.BeginInvoke(
Function()
barcodeReader.DecodeAsync(inputImage)
End Function)
In C#:
Deployment.Current.Dispatcher.BeginInvoke(() =>
{
barcodeReader.DecodeAsync(aBitmapSourceObject);
});
The ReaderDecodeArgs class is passed as a parameter in the DecodeComplete event. The class contains the following information:
FilteredImage – an image with the recognized barcode symbols
SymbolFound – a bool argument indicating whether a barcode is found
Symbology – the symbology of the decoded barcode symbol
Value – the encoded string information in the barcode symbol
The types, for which the Barcode Reader will search, can be optionally specified using the Symbology enumeration.
Unspecified – symbology is not specified, search for all
Code39
Code39Ext – Code 39 Extended.
Code128
Ean13
Ean8
UpcA
UpcE
EanUpc – family of UPC-A, UPC-E, EAN-8, EAN-13 symbologies.
Interleaved2Of5
QRCode
Linear (Code39Ext | Code128 | EanUpc | Interleaved2Of5) – all supported linear symbologies.
All (Linear | QRCode) – all supported symbologies.
The following example specifies the Code39 and Code128 enums:
In Visual Basic:
Dim symbologyTypes As Symbology = Symbology.Code39 Or Symbology.Code128
barcodeReader.Decode(aBitmapSourceObject, symbologyTypes)
In C#:
Symbology symbologyTypes = Symbology.Code39 | Symbology.Code128;
barcodeReader.Decode(aBitmapSourceObject, symbologyTypes);
Below is the complete code used in the examples. The sample demonstrates how to instantiate a new Barcode Reader object and configuring its properties. The Barcode Reader in the sample will scan up to three Code 39 or Code 128 symbols with a minimum size of 200 pixels, with horizontal orientation.
In Visual Basic:
Private Sub ButtonDecode_Click(sender As Object, e As RoutedEventArgs)
Dim barcodeReader As New BarcodeReader()
barcodeReader.MaxNumberOfSymbolsToRead = 3
barcodeReader.MinSymbolSize = 200
barcodeReader.BarcodeOrientation = SymbolOrientation.Horizontal
AddHandler barcodeReader.DecodeComplete, New EventHandler(Of ReaderDecodeArgs)(AddressOf BarcodeReader_DecodeComplete)
Dim symbologyTypes As Symbology = Symbology.Code39 Or Symbology.Code128
barcodeReader.Decode(aBitmapSourceObject, symbologyTypes)
End Sub
Private Sub BarcodeReader_DecodeComplete(sender As Object, e As ReaderDecodeArgs)
If e.SymbolFound Then
Dim result As String = e.Symbology & “ “ & e.Value
End If
End Sub
In C#:
void ButtonDecode_Click(object sender, RoutedEventArgs e)
{
BarcodeReader barcodeReader = new BarcodeReader();
barcodeReader.MaxNumberOfSymbolsToRead = 3;
barcodeReader.MinSymbolSize = 200;
barcodeReader.BarcodeOrientation = SymbolOrientation.Horizontal;
barcodeReader.DecodeComplete += new EventHandler<ReaderDecodeArgs>(BarcodeReader_DecodeComplete);
Symbology symbologyTypes = Symbology.Code39 | Symbology.Code128;
barcodeReader.Decode(aBitmapSourceObject, symbologyTypes);
}
void BarcodeReader_DecodeComplete(object sender, ReaderDecodeArgs e)
{
if(e.SymbolFound)
{
string result = e.Symbology + “ “ + e.Value;
}
}
Related Topic