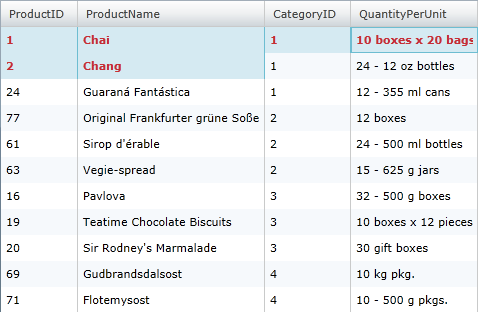
Please note that this control has been retired and is now obsolete to the XamDataGrid control, and as such, we recommend migrating to that control. It will not be receiving any new features, bug fixes, or support going forward. For help or questions on migrating your codebase to the XamDataGrid, please contact support.
This topic demonstrates how you can verify that the end-user cell selection can be pasted correctly in a Microsoft® Excel® worksheet. Only a rectangular cells selection is accepted as a valid region.
The following table lists the topics required as a prerequisite to understanding this topic.
This topic contains the following sections:
In an Excel document, if you select multiple data cells that do not form a rectangular region and try to copy them, a message will be displayed that this is not possible. A rectangular selection in the only valid cell selection for copying.
In the xamGrid control you can check the cell selection that will be copied and pasted. To do this, use the ClipboardCopyingEventArgs object’s ValidateSelectedRectangle method.
The following procedure demonstrates how you can check the cell selection. If the cell selection is not valid, the selected cells are marked in red. A message is displayed that the region is not a rectangle and further copy actions are cancelled.
The following screenshot is a preview of the final result.
To complete the procedure, you need to include the DataUtil class provided for you as a sample data source in your project.
This topic takes you step-by-step toward verifying a rectangular cell selection in the xamGrid control. The following is a conceptual overview of the process:
The following steps demonstrate how to implement the verification of the rectangular region.
Add a xamGrid control to your application and bind in to data source. The verification for a rectangular cell selection is performed in the ClipboardCopying event handler.
In XAML:
<ig:XamGrid x:Name="dataGrid"
ClipboardCopying="dataGrid_ClipboardCopying">
<!-- Add more code here -->
</ig:XamGrid>
Enable the xamGrid copy feature for selected cells so the end user can copy muliple cells content.
In XAML:
<ig:XamGrid.ClipboardSettings>
<ig:ClipboardSettings AllowCopy="True"
CopyOptions="ExcludeHeaders"
CopyType="SelectedCells"
AllowPaste="True"/>
</ig:XamGrid.ClipboardSettings>
The multiple cell selection is enabled in the xamGrid so the end user can select cells to copy.
In XAML:
<ig:XamGrid.SelectionSettings>
<ig:SelectionSettings
CellClickAction="SelectCell"
CellSelection="Multiple" />
</ig:XamGrid.SelectionSettings>
The created Style colors the cell foreground and border in red and sets bolded font weight to the cell text. This way the end user is notified which cells are included in the invalid selection.
In XAML:
<Style x:Key="InvalidSelectionCellStyle"
TargetType="ig:CellControl">
<Setter Property="Foreground" Value="#FFc62d36" />
<Setter Property="BorderBrush" Value="#FFc62d36" />
<Setter Property="BorderThickness" Value="1" />
<Setter Property="FontWeight" Value="Bold" />
</Style>
A check is performed if the cell selection is valid. If the selection is not rectangular, the selected cells are marked in red. Further copy events are cancelled.
In C#:
private void dataGrid_ClipboardCopying(object sender, ClipboardCopyingEventArgs e)
{
// Check if the selected region of cells is valid for pasting
bool isValidSelection = e.ValidateSelectedRectangle();
if (!isValidSelection)
{
foreach (CellBase cell in e.SelectedItems)
{
// Set the new style to the cells that will be copied
cell.Style = this.LayoutRoot.Resources["InvalidSelectionCellStyle"] as Style;
}
// Cancel the copying event if the selected region of cells is not rectangular
e.Cancel = true;
}
}
In Visual Basic:
Private Sub dataGrid_ClipboardCopying(sender As System.Object, e As Infragistics.Controls.Grids.ClipboardCopyingEventArgs)
' Check if the selected region of cells is valid for pasting
Dim isValidSelection As Boolean = e.ValidateSelectedRectangle()
If Not isValidSelection Then
For Each cell As CellBase In e.SelectedItems
' Set the new style to the cells that will be copied
cell.Style = TryCast(Me.LayoutRoot.Resources("InvalidSelectionCellStyle"), Style)
Next
' Cancel the copying event if the selected region of cells is not rectangular
e.Cancel = True
End If
End Sub
The following topics provide additional information related to this topic.