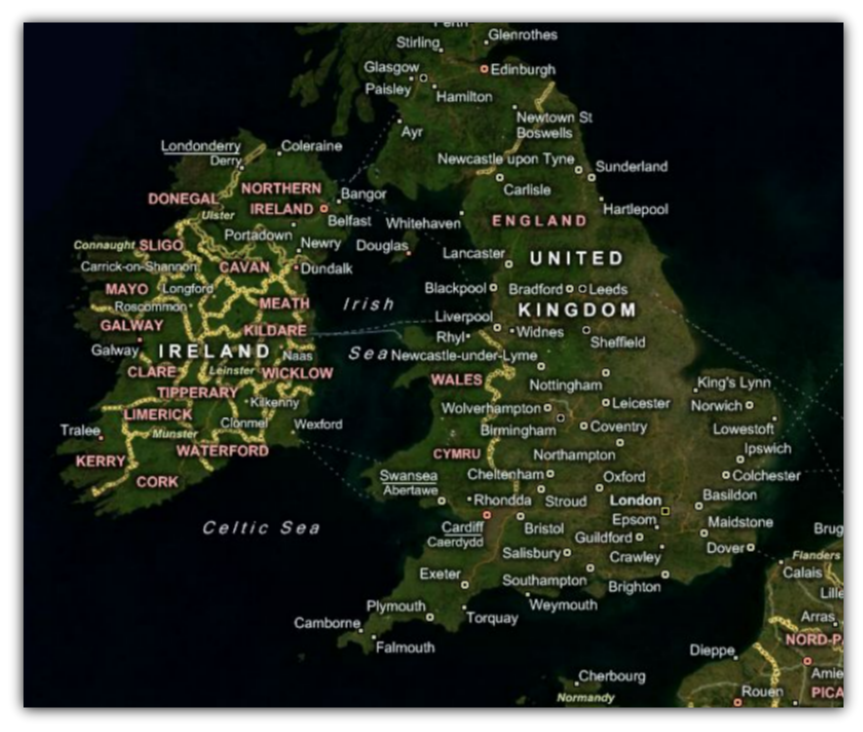
The xamMap™ control supports a number of geo-imagery sources which are listed in the Supported Geo-Imagery section. This topic will focus only on using Bing Maps as the geo-imagery data source. The xamMap control’s MapTileSource property is used with the Microsoft’s Bing Maps imagery service to acquire geo-imagery data. Because the Bing Maps is a licensed web mapping service from Microsoft, you will need to get your own key for Bing Maps Key from Microsoft’s website before you can continue following steps in this topic.
You will add Bing Maps geo-imagery as the data source to the xamMap control and initialize the control’s window map coordinates.
The following set of instructions assumes that you have already set up your project for the xamMap control and that you have your own key for Bing Maps imagery service.
Create a Microsoft® WPF® project.
Add the following NuGet package reference to your project:
Infragistics.WPF.Controls.Maps.XamMap
For more information on setting up the NuGet feed and adding NuGet packages, you can take a look at the following documentation: NuGet Feeds.
Add the following namespaces declaration for the xamMap control.
In XAML:
xmlns:ig="http://schemas.infragistics.com/xaml"
In Visual Basic:
Imports Infragistics.Controls.Maps Imports Infragistics
In C#:
using Infragistics.Controls.Maps; using Infragistics;
Add the xamMap control to your main XAML file:
In XAML:
<ig:XamMap x:Name="igMap"> <!-- NOTE: You can add more Map Layer objects here, e.g. with Shapefile --> </ig:XamMap>
Note: The following steps are necessary to set up the xamMap control to receive geo-imagery map tiles from the Bing Maps imagery service.
Right-click on your application project and select the Add Service Reference menu item.
Click on the Advanced… button in Add Service Reference window.
Check “Generate asynchronous operations” in the Client section of the Service Reference Settings window.
Click on the Add Web Reference… button in Service Reference Settings window.
Enter the following address in the URL textbox and click on the Go button:
Enter BingImageryServiceReference in the Web Reference Name textbox and click on the Add Reference button.
In the constructor of UserControl, add handler for the UserControl’s Loaded event and implement it.
In Visual Basic:
Private Sub UserControl_Loaded(ByVal sender As System.Object, ByVal e As System.Windows.RoutedEventArgs) InitImageryService() InitMapCoordinates() End Sub
In C#:
private void UserControl_Loaded(object sender, RoutedEventArgs e) { InitImageryService(); InitMapCoordinates(); }
Add the following method that will initialize the Bing Maps imagery service to request geo-imagery map data.
In Visual Basic:
Private Sub InitImageryService() Dim mapImgRequest As New BingImageryServiceReference.ImageryMetadataRequest() ' NOTE: You need provide your own key for Bing Maps mapImgRequest.Credentials = New BingImageryServiceReference.Credentials() mapImgRequest.Credentials.ApplicationId = "BING_MAPS_SECRET_KEY" mapImgRequest.Style = BingImageryServiceReference.MapStyle.AerialWithLabels ' create asynchronous Bing Maps service to handle geo-imagery requests Dim imgService As New BingImageryServiceReference.ImageryServiceClient("BasicHttpBinding_IImageryService") AddHandler imgService.GetImageryMetadataCompleted, AddressOf OnImageryServiceGetImageryMetadataCompleted imgService.GetImageryMetadataAsync(mapUriRequest) End Sub
In C#:
private void InitImageryService() { BingImageryServiceReference.ImageryMetadataRequest mapImgRequest = new BingImageryServiceReference.ImageryMetadataRequest(); // NOTE: You need provide your own key for Bing Maps mapImgRequest.Credentials = new BingImageryServiceReference.Credentials(); mapImgRequest.Credentials.ApplicationId = "BING_MAPS_SECRET_KEY"; mapImgRequest.Style = BingImageryServiceReference.MapStyle.AerialWithLabels; // create asynchronous Bing Maps service to handle geo-imagery requests BingImageryServiceReference.ImageryServiceClient imgService = new BingImageryServiceReference.ImageryServiceClient("BasicHttpBinding_IImageryService"); imgService.GetImageryMetadataCompleted += OnImageryServiceGetImageryMetadataCompleted; imgService.GetImageryMetadataAsync(mapImgRequest); }
Add the following method to handle geo-imagery requests and to display geo-imagery on the xamMap control.
In Visual Basic:
Private Sub OnImageryServiceGetImageryMetadataCompleted(ByVal sender As Object, ByVal e As BingImageryServiceReference.GetImageryMetadataCompletedEventArgs) Dim result As BingImageryServiceReference.ImageryMetadataResult = e.Result.Results(0) Dim source As New Infragistics.Controls.Maps.BingMapsTileSource() source.TilePath = result.ImageUri source.SubDomains = New ObservableCollection(Of String)(result.ImageUriSubdomains) igMap.MapTileSource = source End Sub
In C#:
private void OnImageryServiceGetImageryMetadataCompleted(object sender, BingImageryServiceReference.GetImageryMetadataCompletedEventArgs e) { BingImageryServiceReference.ImageryMetadataResult result = e.Result.Results[0]; igMap.MapTileSource = newInfragistics.Controls.Maps.BingMapsTileSource() { TilePath = result.ImageUri, SubDomains = new ObservableCollection<string>(result.ImageUriSubdomains) }; }
Add the following method to initialize the xamMap control’s world map coordinates.
In Visual Basic:
Private Sub InitMapCoordinates() ' define world dimensions Dim worldTopLeft As Point = New Point(-180, 90) Dim worldBottomRight As Point = New Point(180, -90) ' Convert Geodetic to Cartesian coordinates Dim winTopLeft As Point = Me.xamMap.MapProjection.ProjectToMap(worldTopLeft) Dim winBottomRight As Point = Me.xamMap.MapProjection.ProjectToMap(worldBottomRight) ' Create Rect structure the map control's WindowRect and WorldRect Dim winRect As New Rect() winRect.X = Math.Min(winTopLeft.X, winBottomRight.X) winRect.Y = Math.Min(winTopLeft.Y, winBottomRight.Y) winRect.Width = Math.Abs(winTopLeft.X - winBottomRight.X) winRect.Height = Math.Abs(winTopLeft.Y - winBottomRight.Y) Me.igMap.IsAutoWorldRect = False Me.igMap.WindowZoomMaximum = 80 ' Change the map control's WindowRect and WorldRect Me.igMap.WorldRect = winRect Me.igMap.WindowRect = winRect End Sub
In C#:
private void InitMapCoordinates() { // define world dimensions Point worldTopLeft = new Point(-180, 90); Point worldBottomRight = new Point(180, -90); // Convert Geodetic to Cartesian coordinates Point winTopLeft = this.igMap.MapProjection.ProjectToMap(worldTopLeft); Point winBottomRight = this.igMap.MapProjection.ProjectToMap(worldBottomRight); // Create Rect structure the map control's WindowRect and WorldRect Rect winRect = new Rect() { X = Math.Min(winTopLeft.X, winBottomRight.X), Y = Math.Min(winTopLeft.Y, winBottomRight.Y), Width = Math.Abs(winTopLeft.X - winBottomRight.X), Height = Math.Abs(winTopLeft.Y - winBottomRight.Y) }; this.igMap.IsAutoWorldRect = false; this.igMap.WindowZoomMaximum = 80; // Change the map control's WindowRect and WorldRect this.igMap.WindowRect = this.igMap.WorldRect = winRect; }
Save and run your project. The xamMap control will load and display the geo-imagery data from Bing Maps source. The following screenshot shows the result.