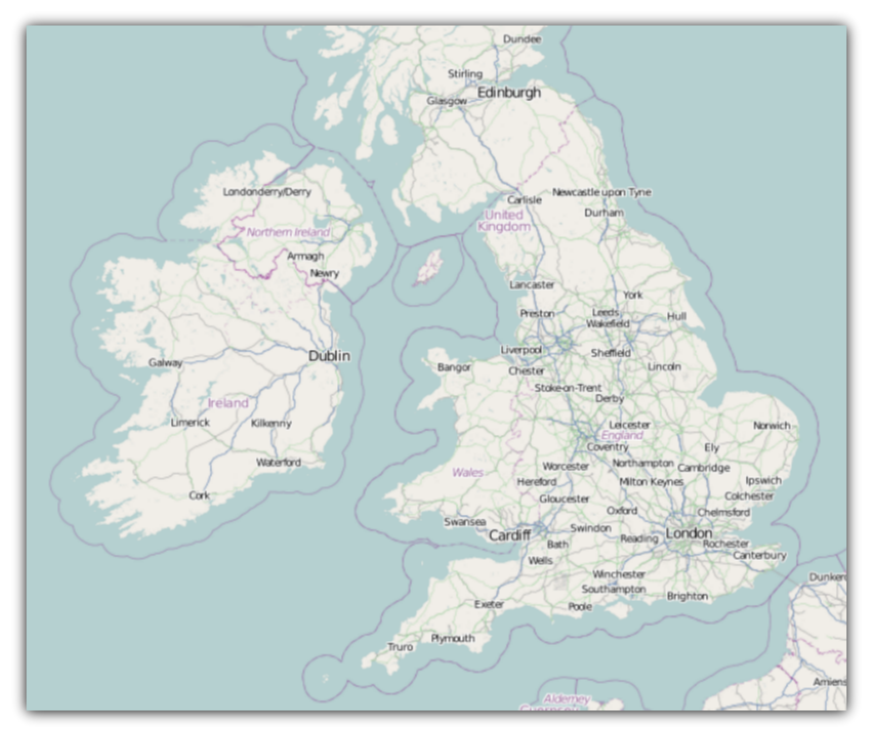
The xamMap™ control’s MapTileSource property is used to configure the source from which geo-imagery is loaded. Please refer to the Supported Geo-Imagery section for a complete list of supported geo-imagery source. However this section will focus only on OpenStreetMap as the geo-imagery source. The Open Street Maps web mapping service is not licensed so you do not provide any license keys for it to work with the xamMap control.
You will add Open Street Maps as geo-imagery source to the xamMap control and set its world map coordinates.
The following set of instructions assumes that you have already set up your WPF project for the xamMap control.
Create a Microsoft® WPF® project.
Add the following NuGet package reference to your project:
Infragistics.WPF.Controls.Maps.XamMap
For more information on setting up the NuGet feed and adding NuGet packages, you can take a look at the following documentation: NuGet Feeds.
Add the following namespaces declaration for the xamMap control.
In XAML:
xmlns:igMap="http://schemas.infragistics.com/xaml"
In Visual Basic:
Imports Infragistics.Controls.Maps Imports Infragistics
In C#:
using Infragistics.Controls.Maps; using Infragistics;
Add the XamMap control with appropriate projection type
In XAML:
<igMap:XamMap x:Name="xamMap" > <!-- TODO: Add Open Street Maps as geo-imagery data source --> </igMap:XamMap>
Set the MapTileSource property to OpenStreetMapTileSource.
In XAML:
<igMap:XamMap.MapTileSource > <igMap:OpenStreetMapTileSource/> </igMap:XamMap.MapTileSource>
In code behind, add event handler for the User Control object’s Loaded event.
In Visual Basic:
Public Sub New() InitializeComponent() AddHandler Me.Loaded, AddressOf UserControl_Loaded End Sub
In C#:
public MainPage() { InitializeComponent(); this.Loaded += new RoutedEventHandler(UserControl_Loaded); }
Implement the event handler for the UserControl’s Loaded event.
In Visual Basic:
Private Sub UserControl_Loaded(ByVal sender As System.Object, ByVal e As System.Windows.RoutedEventArgs) InitMapCoordinates() End Sub
In C#:
private void UserControl_Loaded(object sender, RoutedEventArgs e) { InitMapCoordinates(); }
Add the following method to initialize the xamMap control’s world map coordinates.
In Visual Basic:
Private Sub InitMapCoordinates() ' Convert define world dimensions Dim worldTopLeft As Point = New Point(-180, 90) Dim worldBottomRight As Point = New Point(180, -90) ' Convert Geodetic to Cartesian coordinates Dim winTopLeft As Point = Me.xamMap.MapProjection.ProjectToMap(worldTopLeft) Dim winBottomRight As Point = Me.xamMap.MapProjection.ProjectToMap(worldBottomRight) ' Create Rect structure the map control's WindowRect and WorldRect Dim winRect As New Rect() winRect.X = Math.Min(winTopLeft.X, winBottomRight.X) winRect.Y = Math.Min(winTopLeft.Y, winBottomRight.Y) winRect.Width = Math.Abs(winTopLeft.X - winBottomRight.X) winRect.Height = Math.Abs(winTopLeft.Y - winBottomRight.Y) Me.xamMap.IsAutoWorldRect = False Me.xamMap.WindowZoomMaximum = 80 ' Change the map control's WindowRect and WorldRect Me.xamMap.WorldRect = winRect Me.xamMap.WindowRect = winRect End Sub
In C#:
private void InitMapCoordinates() { // define world dimensions Point worldTopLeft = new Point(-180, 90); Point worldBottomRight = new Point(180, -90); // Convert Geodetic to Cartesian coordinates Point winTopLeft = this.xamMap.MapProjection.ProjectToMap(worldTopLeft); Point winBottomRight = this.xamMap.MapProjection.ProjectToMap(worldBottomRight); // Create Rect structure the map control's WindowRect and WorldRect Rect winRect = new Rect() { X = Math.Min(winTopLeft.X, winBottomRight.X), Y = Math.Min(winTopLeft.Y, winBottomRight.Y), Width = Math.Abs(winTopLeft.X - winBottomRight.X), Height = Math.Abs(winTopLeft.Y - winBottomRight.Y) }; this.xamMap.IsAutoWorldRect = false; this.xamMap.WindowZoomMaximum = 80; // Change the map control's WindowRect and WorldRect this.xamMap.WindowRect = this.xamMap.WorldRect = winRect; }
Run the application. The xamMap control will load and display the geo-imagery data from Open Street Maps source. The following screenshot shows the result.