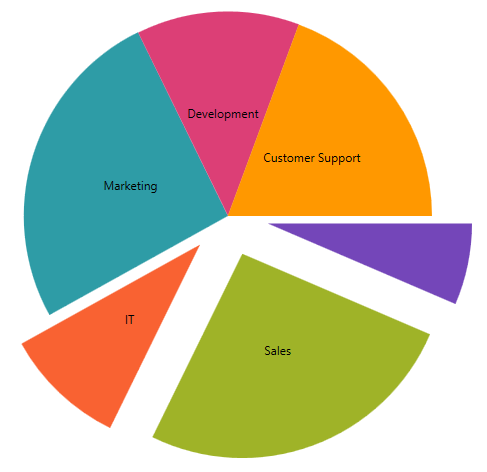
This topic demonstrates how to implement the explosion behavior of the XamPieChart™ control. At the end of the topic, a complete code sample is provided.
The topic is organized as follows:
The XamPieChart control supports explosion of individual pie slices as well as a SliceClick event that allows you to modify selection states and implement custom logic.
Figure 1: The XamPieChart control as implemented by the sample code
This article assumes you have already read the Data Binding topic, and uses the code therein as a starting point.
Configuring the respective properties and event handler
Implementing the event handler
(Optional) Verifying the result
Configure the respective properties and event handler .
Taking code from the Data Binding topic as a starting point, enable explosion by setting the AllowSliceExplosion property to True and configure pieChart_SliceClick as the event handler for mouse clicks:
In XAML:
<ig:XamPieChart Name="pieChart" AllowSliceExplosion="True" SliceClick="pieChart_SliceClick" />
Implement the event handler .
On SliceClick, toggle the explosion states of the slice.
In C#:
private void pieChart_SliceClick(object sender, Infragistics.XamarinForms.Controls.Charts.SliceClickEventArgs e) { e.IsExploded = !e.IsExploded; }
(Optional) Verify the result .
To verify the result, run your application. The Pie Chart control will now respond to SliceClick events by selecting and exploding the appropriate slice outward. A list of currently selected slices will also be maintained in the upper left corner. (Figure 1, above)
The following code listings contain the full example implemented in context.
<?xml version="1.0" encoding="UTF-8"?> <ContentView xmlns="http://xamarin.com/schemas/2014/forms" xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml" xmlns:ig="clr-namespace:Infragistics.XamarinForms.Controls.Charts;assembly=Infragistics.XF.Charts" x:Class="XamPieChart_SelectAndExplodeView"> <Grid> <ig:XamPieChart x:Name="pieChart" LabelMemberPath="Label" ValueMemberPath="Value" AllowSliceExplosion="True" LabelsPosition="BestFit" ItemsSource="{Binding Data}" SliceClick="pieChart_SliceClick"> </ig:XamPieChart> </Grid> </ContentView>
using Infragistics.XamarinForms.Controls.Charts; using System.Collections.ObjectModel; using Xamarin.Forms; namespace XamPieChart_SelectAndExplode { public partial class Explosion : ContentView { public Data Data { get; set; } public Explosion() { InitializeComponent(); Data = new Data(); this.BindingContext = this; } private void pieChart_SliceClick(object sender, SliceClickEventArgs e) { e.IsExploded = !e.IsExploded; } } public class DataItem { public string Label { get; set; } public double Value { get; set; } } public class Data : ObservableCollection<DataItem> { public Data() { Add(new DataItem { Label = "Administration", Value = 20 }); Add(new DataItem { Label = "Sales", Value = 80 }); Add(new DataItem { Label = "IT", Value = 30 }); Add(new DataItem { Label = "Marketing", Value = 80 }); Add(new DataItem { Label = "Development", Value = 40 }); Add(new DataItem { Label = "Customer Support", Value = 60 }); } } }