계층적 Data Grid 개요 및 구성
Ignite UI for React 계층적 데이터 그리드는 계층적 테이블 형식 데이터를 표시하고 조작하는 데 사용됩니다. 아주 적은 코드로 데이터를 빠르게 바인딩하거나 다양한 이벤트를 사용하여 다양한 동작을 사용자 지정할 수 있습니다. 이 구성 요소는 데이터 선택, Excel 스타일 필터링, 정렬, 페이징, 템플릿, 열 이동, 열 고정, Excel 및 CSV로 내보내기 등과 같은 다양한 기능을 제공합니다. 계층적 그리드는 플랫 그리드 컴포넌트를 기반으로 하며, 사용자가 부모 그리드의 행을 확장하거나 축소할 수 있도록 하여 기능을 확장하고, 더 자세한 정보가 필요할 때 해당 자식 그리드를 표시합니다.
React Hierarchical Data Grid Example
이 React 표 예제에서는 사용자가 계층적 데이터 집합을 시각화하고 셀 템플릿을 사용하여 다른 시각적 구성 요소를 추가하는 방법을 확인할 수 있습니다.
Getting Started with Ignite UI for React Hierarchical Grid
Dependencies
React 계층적 그리드를 시작하려면 먼저 and igniteui-react-grids
패키지를 설치해야 igniteui-react
합니다.
npm install --save igniteui-react
npm install --save igniteui-react-grids
그리드를 사용하려면 다음 가져오기도 포함해야 합니다.
import "igniteui-react-grids/grids/combined.js";
해당 스타일도 참조해야 합니다. 테마 중 하나에 대해 밝거나 어두운 옵션을 선택하고 프로젝트 구성에 따라 테마를 가져올 수 있습니다.
import 'igniteui-react-grids/grids/themes/light/bootstrap.css'
계층적 그리드의 모양을 사용자 지정하는 방법에 대한 자세한 내용은 스타일 섹션을 참조하십시오.
Using the React Hierarchical Data Grid
Data Binding
IgrHierarchicalGrid는 IgrGrid에서 파생되며 대부분의 기능을 공유합니다. 주요 차이점은 여러 수준의 계층 구조를 정의할 수 있다는 것입니다. IgrRowIsland 라는 IgrHierarchicalGrid 정의 내에서 별도의 태그를 통해 구성됩니다. IgrRowIsland 구성 요소는 특정 수준의 각 자식 그리드에 대한 구성을 정의합니다. 수준당 여러 행 고립영역도 지원됩니다. 계층적 그리드는 데이터에 바인딩하는 두 가지 방법을 지원합니다.
Using hierarchical data
애플리케이션이 전체 계층 데이터를 개체의 하위 배열을 참조하는 개체 배열로 로드하는 경우 계층 그리드는 이를 읽고 자동으로 바인딩하도록 구성할 수 있습니다. 다음은 적절하게 구조화된 계층적 데이터 원본의 예입니다.
export const singers = [{
"Artist": "Naomí Yepes",
"Photo": "assets/images/hgrid/naomi.png",
"Debut": "2011",
"Grammy Nominations": 6,
"Grammy Awards": 0,
"Tours": [{
"Tour": "Faithful Tour",
"Started on": "Sep-12",
"Location": "Worldwide",
"Headliner": "NO",
"Toured by": "Naomí Yepes"
}],
"Albums": [{
"Album": "Dream Driven",
"Launch Date": new Date("August 25, 2014"),
"Billboard Review": "81",
"US Billboard 200": "1",
"Artist": "Naomí Yepes",
"Songs": [{
"No.": "1",
"Title": "Intro",
"Released": "*",
"Genre": "*",
"Album": "Dream Driven"
}]
}]
}];
각 IgrRowIsland는 자식 데이터를 보유하는 속성의 키를 지정해야 합니다.
<IgrHierarchicalGrid data={singers} autoGenerate="true">
<IgrRowIsland childDataKey="Albums" autoGenerate="true">
<IgrRowIsland childDataKey="Songs" autoGenerate="true">
</IgrRowIsland>
</IgrRowIsland>
<IgrRowIsland childDataKey="Tours" autoGenerate="true">
</IgrRowIsland>
</IgrHierarchicalGrid>
[!NOTE] Note that instead of
data
the user configures only thechildDataKey
that the IgrHierarchicalGrid needs to read to set the data automatically.
Using Load-On-Demand
대부분의 응용 프로그램은 초기에 가능한 한 적은 데이터를 로드하도록 설계되어 로드 시간이 더 빨라집니다. 이러한 경우 사용자가 만든 서비스가 요청 시 데이터를 제공할 수 있도록 IgrHierarchicalGrid를 구성할 수 있습니다.
import { getData } from "./remoteService";
export default function Sample() {
const hierarchicalGrid = useRef<IgrHierarchicalGrid>(null);
function gridCreated(
rowIsland: IgrRowIsland,
event: IgrGridCreatedEventArgs,
_parentKey: string
) {
const context = event.detail;
const dataState = {
key: rowIsland.childDataKey,
parentID: context.parentID,
parentKey: _parentKey,
rootLevel: false,
};
context.grid.isLoading = true;
getData(dataState).then((data: any[]) => {
context.grid.isLoading = false;
context.grid.data = data;
context.grid.markForCheck();
});
}
useEffect(() => {
hierarchicalGrid.current.isLoading = true;
getData({ parentID: null, rootLevel: true, key: "Customers" }).then(
(data: any) => {
hierarchicalGrid.current.isLoading = false;
hierarchicalGrid.current.data = data;
hierarchicalGrid.current.markForCheck();
}
);
}, []);
return (
<IgrHierarchicalGrid
ref={hierarchicalGrid}
primaryKey="customerId"
autoGenerate="true"
height="600px"
width="100%"
>
<IgrRowIsland
childDataKey="Orders"
primaryKey="orderId"
autoGenerate="true"
gridCreated={(
rowIsland: IgrRowIsland,
e: IgrGridCreatedEventArgs
) => gridCreated(rowIsland, e, "Customers")}
>
<IgrRowIsland
childDataKey="Details"
primaryKey="productId"
autoGenerate="true"
gridCreated={(
rowIsland: IgrRowIsland,
e: IgrGridCreatedEventArgs
) => gridCreated(rowIsland, e, "Orders")}
></IgrRowIsland>
</IgrRowIsland>
</IgrHierarchicalGrid>
);
}
const URL = `https://data-northwind.indigo.design/`;
export function getData(dataState: any): any {
return fetch(buildUrl(dataState))
.then((result) => result.json());
}
function buildUrl(dataState: any) {
let qS = "";
if (dataState) {
if (dataState.rootLevel) {
qS += `${dataState.key}`;
} else {
qS += `${dataState.parentKey}/${dataState.parentID}/${dataState.key}`;
}
}
return `${URL}${qS}`;
}
Hide/Show row expand indicators
행을 확장하기 전에 자식이 있는지 여부에 대한 정보를 제공하는 방법이 있는 경우 input 속성을 사용할 HasChildrenKey
수 있습니다. 이렇게 하면 데이터 개체에서 확장 표시기를 표시해야 하는지 여부를 나타내는 부울 속성을 제공할 수 있습니다.
<IgrHierarchicalGrid data={data} primaryKey="ID" hasChildrenKey="hasChildren">
</IgrHierarchicalGrid>
속성을 설정할 HasChildrenKey
필요는 없습니다. 제공하지 않으면 각 행에 확장 표시기가 표시됩니다.
또한 머리글 확장/축소 표시기를 ShowExpandAll
표시하거나 숨기려면 속성을 사용할 수 있습니다. 이 UI는 성능상의 이유로 기본적으로 사용하지 않도록 설정되며, 대용량 데이터가 있는 그리드 또는 요청 시 로드가 있는 그리드에서는 사용하지 않는 것이 좋습니다.
특징
그리드 기능은 IgrRowIsland 마크업을 통해 활성화하고 구성할 수 있으며, 생성된 모든 그리드에 적용됩니다. 행 섬 인스턴스를 통해 런타임에 옵션을 변경하면 생성된 각 그리드에 대해 옵션이 변경됩니다.
<IgrHierarchicalGrid data={localData} autoGenerate="false"
allowFiltering='true' height="600px" width="800px">
<IgrColumn field="ID" pinned="true" filterable="true"></IgrColumn>
<IgrColumnGroup header="Information">
<IgrColumn field="ChildLevels"></IgrColumn>
<IgrColumn field="ProductName" hasSummary="true"></IgrColumn>
</IgrColumnGroup>
<IgrRowIsland childDataKey="childData" autoGenerate="false" rowSelection="multiple">
<IgrColumn field="ID" hasSummary="true" dataType="number"></IgrColumn>
<IgrColumnGroup header="Information2">
<IgrColumn field="ChildLevels"></IgrColumn>
<IgrColumn field="ProductName"></IgrColumn>
</IgrColumnGroup>
<IgrPaginator perPage={5}></IgrPaginator>
<IgrRowIsland>
<IgrPaginator></IgrPaginator>
</IgrHierarchicalGrid>
다음 그리드 기능은 그리드 수준별로 작동합니다. 즉, 각 그리드 인스턴스가 나머지 그리드와 독립적으로 해당 기능을 관리합니다.
- 정렬
- 필터링
- 페이징
- 다중 열 헤더
- 숨김
- 고정
- 움직이는
- 요약
- 찾다
Selection(선택) 및 Navigation(탐색) 기능은 전체 계층적 그리드(Hierarchical Grid)에 대해 전역적으로 작동합니다
- 선택 선택에서는 두 개의 서로 다른 하위 그리드에 대해 선택한 셀이 동시에 표시되는 것을 허용하지 않습니다.
- 탐색 위/아래로 탐색할 때 다음/이전 요소가 하위 그리드인 경우 관련 하위 그리드에서 탐색이 계속되며 관련 셀이 선택되고 초점이 맞춰진 것으로 표시됩니다. 하위 셀이 현재 표시되는 뷰 포트 외부에 있는 경우 선택한 셀이 항상 표시되도록 뷰로 스크롤됩니다.
Collapse All Button
계층적 그리드를 사용하면 사용자는 왼쪽 상단 모서리에 있는 "모두 축소" 버튼을 눌러 현재 확장된 모든 행을 편리하게 축소할 수 있습니다. 또한 다른 그리드를 포함하고 계층적 그리드 자체인 모든 하위 그리드에도 다음과 같은 버튼이 있습니다. 이 방법으로 사용자는 계층 구조에서 지정된 그리드만 축소할 수 있습니다.
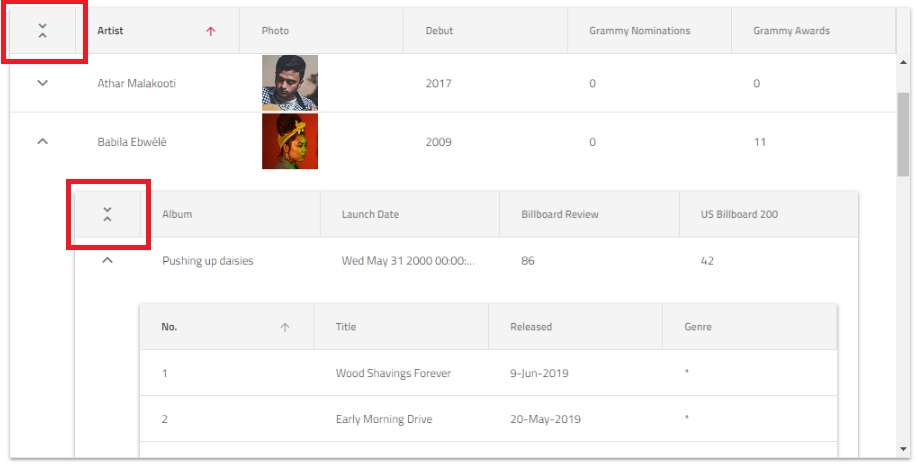
Styling
사전 정의된 테마 외에도 사용 가능한 CSS 속성 중 일부를 설정하여 그리드를 추가로 사용자 정의할 수 있습니다. 헤더 배경과 텍스트 색상을 변경하려면 먼저 그리드에 대한 클래스를 설정해야 합니다.
<IgrHierarchicalGrid className="grid"></IgrHierarchicalGrid>
그런 다음 해당 클래스에 대해--header-background
및--header-text-color
CSS 속성을 설정합니다.
.grid {
--header-background: #494949;
--header-text-color: #FFF;
}
Demo
Known Limitations
한정 | 설명 |
---|---|
그룹화 기준 | 그룹화 기준 기능은 계층형 그리드에서 지원되지 않습니다. |
API References
우리 커뮤니티는 활동적이며 항상 새로운 아이디어를 환영합니다.